Convert ISO 8601 date to human readable format
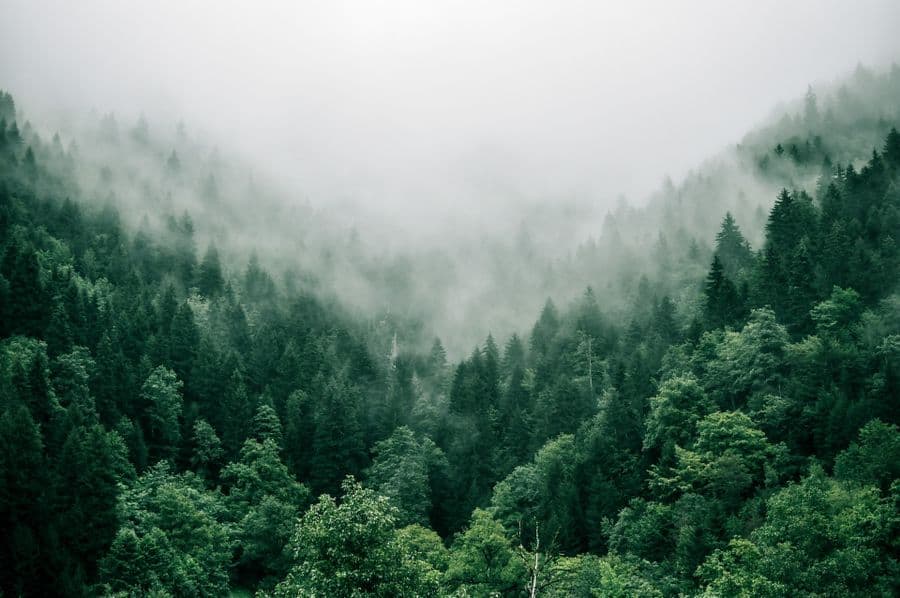
I've come to find that i require the same functions and snippets across many projects. The beauty in reusable code is it's simplicity, and its single output gives it the ability to be added in pretty much any project you get involved with and displaying a human readable date is one i find myself rewriting over and over again.
So either this is for those of you who have found this article through search or myself so i can remember i've already wrote this code.
What is an ISO 8601 datetime format?
ISO 8601 is an international standard for the representation of dates and times. It defines a format for the date and time representation that is unambiguous, easy to read, and widely recognized. The ISO 8601 date format is as follows:
YYYY-MM-DDTHH:mm:ssZ
Where:
YYYY represents the year using four digits.
MM represents the month using two digits (01 for January, 02 for February, etc.).
DD represents the day of the month using two digits (01 for the first day, 02 for the second day, etc.).
T is a separator between the date and time portions of the string.
HH represents the hour using two digits (00 for midnight, 01 for 1am, 02 for 2am, etc.).
mm represents the minute using two digits (00 to 59).
ss represents the second using two digits (00 to 59).
Z represents the time zone offset from UTC, which can be either "Z" for UTC or a numerical offset from UTC (e.g. +05:00 for five hours ahead of UTC).
For example, the ISO 8601 format for March 29, 2023, at 3:30 PM UTC would be:
2023-03-29T15:30:00Z
How to convert it to a date using JavaScript
There are many different ways to convert an ISO 8601 datetime format. You can split the string to remove the last 10 characters, split before 'T'. However depending on your location this many not be in the date format your website or app users are used to using. I like to use toLocaleDateString() which means i can add in the locale used on the page render to show the correct format.
let isoDate = "2023-03-23T00:26:00Z"; // Capture the datetime string.
var readableDate = new Date(isoDate); // Convert the isoDate into a new Date string.
readableDate.toLocaleDateString('en-GB'); // If you are from the UK like me it will display the date format as dd/mm/yyyy
readableDate.toLocaleDateString('en-US'); // If you are in the USA it will display the date format as mm/dd/yyyy
I hope the above, although a short tutorial example can help you resolve a common task.
If you have a better solution or one you wish to add to this article to help others please do get in touch.